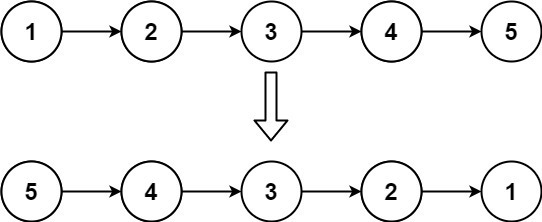
문제 Given the head of a singly linked list, reverse the list, and return the reversed list. 예시 Example 1: Input: head = [1,2,3,4,5] Output: [5,4,3,2,1] Example 2: Input: head = [1,2] Output: [2,1] Example 3: Input: head = [] Output: [] 제약조건 Constraints: The number of nodes in the list is the range [0, 5000]. -5000
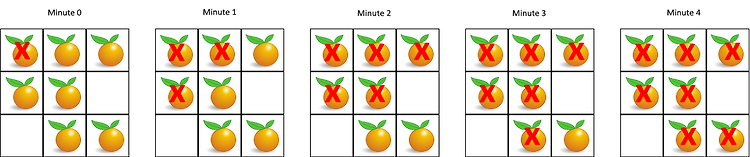
문제 You are given an m x n grid where each cell can have one of three values: 0 representing an empty cell, 1 representing a fresh orange, or 2 representing a rotten orange. Every minute, any fresh orange that is 4-directionally adjacent to a rotten orange becomes rotten. Return the minimum number of minutes that must elapse until no cell has a fresh orange. If this is impossible, return -1. 예시 x..
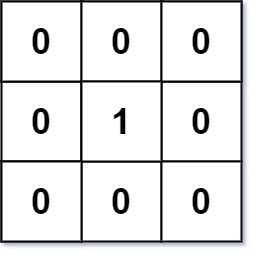
문제 Given an m x n binary matrix mat, return the distance of the nearest 0 for each cell. The distance between two adjacent cells is 1. 예시 Example 1: Input: mat = [[0,0,0],[0,1,0],[0,0,0]] Output: [[0,0,0],[0,1,0],[0,0,0]] Example 2: Input: mat = [[0,0,0],[0,1,0],[1,1,1]] Output: [[0,0,0],[0,1,0],[1,2,1]] 제약 조건 Constraints: m == mat.length n == mat[i].length 1
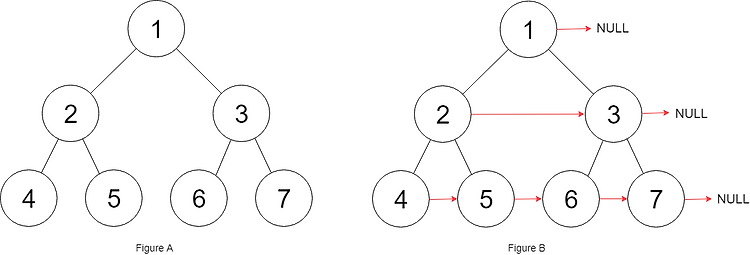
https://leetcode.com/problems/populating-next-right-pointers-in-each-node/ Populating Next Right Pointers in Each Node - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com 문제 You are given a perfect binary tree where all leaves are on the same level, and every parent has two children...
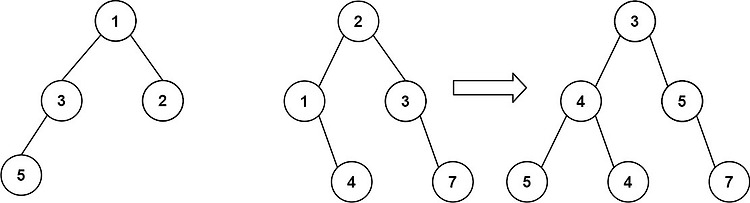
https://leetcode.com/problems/merge-two-binary-trees/ Merge Two Binary Trees - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com 문제 : You are given two binary trees root1 and root2. Imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped w..
문제: 연결 리스트 구현하기 Example 1 : Input ["MyLinkedList", "addAtHead", "addAtTail", "addAtIndex", "get", "deleteAtIndex", "get"] [[], [1], [3], [1, 2], [1], [1], [1]] Output [null, null, null, null, 2, null, 3] Explanation MyLinkedList myLinkedList = new MyLinkedList(); myLinkedList.addAtHead(1); myLinkedList.addAtTail(3); myLinkedList.addAtIndex(1, 2); // linked list becomes 1->2->3 myLinkedList.get(1..
문제 : 정수 배열이 주어졌을 때, 그 중 짝수의 자릿수 nums가 포함된 정수의 수를 반환합니다. Example 1 : Input: nums = [12,345,2,6,7896] Output: 2 Explanation: 12는 2자리(짝수 자릿수)를 포함합니다. 345는 3자리(홀수 자릿수)를 포함합니다. 2는 1자리(홀수 자릿수)를 포함합니다. 6은 1자리(홀수 자릿수)를 포함합니다. 7896은 4자리(짝수 자릿수)를 포함합니다. 따라서 12와 7896만 짝수 자릿수를 포함합니다. Example 1 : Input: nums = [555,901,482,1771] Output: 1 Explanation: 1771에만 짝수 자릿수가 있습니다. 제약 조건 : 1
문제 : Given a binary array nums, return the maximum number of consecutive 1's in the array. 이진 배열이 주어지면 배열 에서 연속되는 최대 수를 반환합니다. Example 1 : Input: nums = [1,1,0,1,1,1] Output: 3 Explanation: The first two digits or the last three digits are consecutive 1s. The maximum number of consecutive 1s is 3. Example 2 : Input: nums = [1,0,1,1,0,1] Output: 2 Constraints : 1
Comment