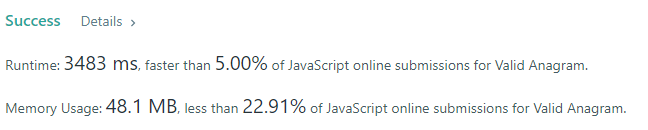
문제 Given two strings s and t, return true if t is an anagram of s, and false otherwise. An Anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once. s와 t 문자열이 주어지면, t가 s의 아나그램일 때 true를 반환하고 아니면 false를 반환한다. 아나그램이란, 단어를 구성하는 알파벳들이 다른 순서로 구성되어있는 또 다른 단어를 말한다. 예시 Example 1: Input: s = "anagram", t = "nagaram" ..

문제 Given an integer array nums, return true if any value appears at least twice in the array, and return false if every element is distinct. 주어진 nums 배열 요소 중 중복되는 값이 있다면 true, 없으면 false를 반환하라. 예시 Example 1: Input: nums = [1,2,3,1] Output: true Example 2: Input: nums = [1,2,3,4] Output: false Example 3: Input: nums = [1,1,1,3,3,4,3,2,4,2] Output: true 제약 조건 Constraints: 1

문제 Design your implementation of the linked list. You can choose to use a singly or doubly linked list. A node in a singly linked list should have two attributes: val and next. val is the value of the current node, and next is a pointer/reference to the next node. If you want to use the doubly linked list, you will need one more attribute prev to indicate the previous node in the linked list. As..
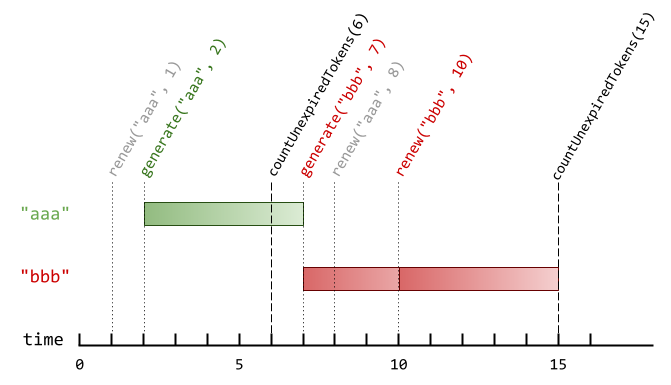
문제 There is an authentication system that works with authentication tokens. For each session, the user will receive a new authentication token that will expire timeToLive seconds after the currentTime. If the token is renewed, the expiry time will be extended to expire timeToLive seconds after the (potentially different) currentTime. Implement the AuthenticationManager class: AuthenticationManag..

문제 You are given a nested list of integers nestedList. Each element is either an integer or a list whose elements may also be integers or other lists. Implement an iterator to flatten it. Implement the NestedIterator class: NestedIterator(List nestedList) Initializes the iterator with the nested list nestedList. int next() Returns the next integer in the nested list. boolean hasNext() Returns tr..

문제 Design a stack that supports push, pop, top, and retrieving the minimum element in constant time. Implement the MinStack class: MinStack() initializes the stack object. void push(int val) pushes the element val onto the stack. void pop() removes the element on the top of the stack. int top() gets the top element of the stack. int getMin() retrieves the minimum element in the stack. You must i..

문제 Design a system that manages the reservation state of n seats that are numbered from 1 to n. Implement the SeatManager class: SeatManager(int n) Initializes a SeatManager object that will manage n seats numbered from 1 to n. All seats are initially available. int reserve() Fetches the smallest-numbered unreserved seat, reserves it, and returns its number. void unreserve(int seatNumber) Unrese..

문제 At a lemonade stand, each lemonade costs $5. Customers are standing in a queue to buy from you and order one at a time (in the order specified by bills). Each customer will only buy one lemonade and pay with either a $5, $10, or $20 bill. You must provide the correct change to each customer so that the net transaction is that the customer pays $5. Note that you do not have any change in hand ..
Comment