1. 문제
2. 예시
3. 제약 조건
4. 해결 코드
https://leetcode.com/problems/add-two-numbers/
Add Two Numbers - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
1. 문제
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
음수가 아닌 두 정수를 나타내는 비어 있지 않은 두 개의 연결 목록이 제공됩니다. 자릿수는 역순으로 저장되며 각 노드는 한 자릿수를 포함합니다. 두 숫자를 더하고 합계를 연결 리스트로 반환합니다.
숫자 0을 제외하고 두 숫자는 선행 0을 포함하지 않는다고 가정할 수 있습니다.
2. 예시
Example 1:
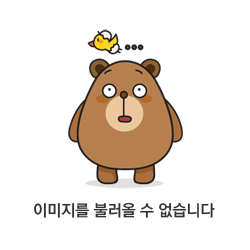
Input: l1 = [2,4,3], l2 = [5,6,4]
Output: [7,0,8]
Explanation: 342 + 465 = 807.
Example 2:
Input: l1 = [0], l2 = [0]
Output: [0]
Example 3:
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9]
Output: [8,9,9,9,0,0,0,1]
3. 제약 조건
Constraints:
- The number of nodes in each linked list is in the range [1, 100].
- 0 <= Node.val <= 9
- It is guaranteed that the list represents a number that does not have leading zeros.
4. 해결 코드
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} l1
* @param {ListNode} l2
* @return {ListNode}
*/
var addTwoNumbers = function(l1, l2) {
return add(l1, l2, 0);
};
const add = (l1, l2, carry) => {
const v1 = (l1 && l1.val) || 0;
const v2 = (l2 && l2.val) || 0;
const sum = v1 + v2 + carry;
const newCarry = Math.floor(sum / 10);
const val = sum % 10;
return (l1 || l2 || carry) ? { val, next: add(l1 && l1.next, l2 && l2.next, newCarry) } : null;
};
javascript
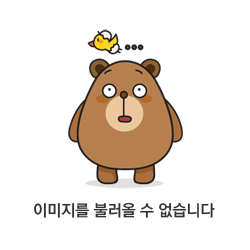
'leetCode' 카테고리의 다른 글
[Medium] 1845. Seat Reservation Manager (0) | 2022.07.26 |
---|---|
[Easy] 860. Lemonade Change (0) | 2022.07.26 |
[Medium] 138. Copy List with Random Pointer (0) | 2022.07.19 |
[Medium] 143. Reorder List (0) | 2022.07.19 |
[Medium] 304. Range Sum Query 2D - Immutable (0) | 2022.07.15 |
Comment