1. 문제
2. 예시
3. 제약 조건
4. 해결 코드
1. 문제
There is an authentication system that works with authentication tokens. For each session, the user will receive a new authentication token that will expire timeToLive seconds after the currentTime. If the token is renewed, the expiry time will be extended to expire timeToLive seconds after the (potentially different) currentTime.
Implement the AuthenticationManager class:
- AuthenticationManager(int timeToLive) constructs the AuthenticationManager and sets the timeToLive.
- generate(string tokenId, int currentTime) generates a new token with the given tokenId at the given currentTime in seconds.
- renew(string tokenId, int currentTime) renews the unexpired token with the given tokenId at the given currentTime in seconds. If there are no unexpired tokens with the given tokenId, the request is ignored, and nothing happens.
- countUnexpiredTokens(int currentTime) returns the number of unexpired tokens at the given currentTime.
Note that if a token expires at time t, and another action happens on time t (renew or countUnexpiredTokens), the expiration takes place before the other actions.
인증 토큰과 함께 작동하는 인증 시스템이 있습니다. 각 세션에 대해 사용자는 현재 시간 이후 timeToLive 초가 만료되는 새 인증 토큰을 받게 됩니다. 토큰이 갱신되면 만료 시간이 (잠재적으로 다른) 현재 시간 이후 만료 시간 ToLive 초로 연장됩니다.
AuthenticationManager 클래스 구현:
AuthenticationManager(int timeToLive)는 AuthenticationManager를 구성하고 TimeToLive를 설정합니다.
generate(string tokenId, intcurrentTime)는 지정된 currentTime(초)에 지정된 tokenId를 사용하여 새 토큰을 생성합니다.
renew(string tokenId, intcurrentTime)는 만료되지 않은 토큰을 지정된 currentTime(초)에 지정된 tokenId로 갱신합니다. 지정된 tokenId를 가진 만료되지 않은 토큰이 없으면 요청이 무시되고 아무 일도 일어나지 않습니다.
만료되지 않은 카운트토큰(intcurrentTime)은 지정된 currentTime에서 만료되지 않은 토큰 수를 반환합니다.
토큰이 t 시간에 만료되고 t 시간에 다른 작업이 발생하는 경우(갱신 또는 countUnexpired).토큰) 만료는 다른 작업보다 먼저 발생합니다.
2. 예시
Example 1:
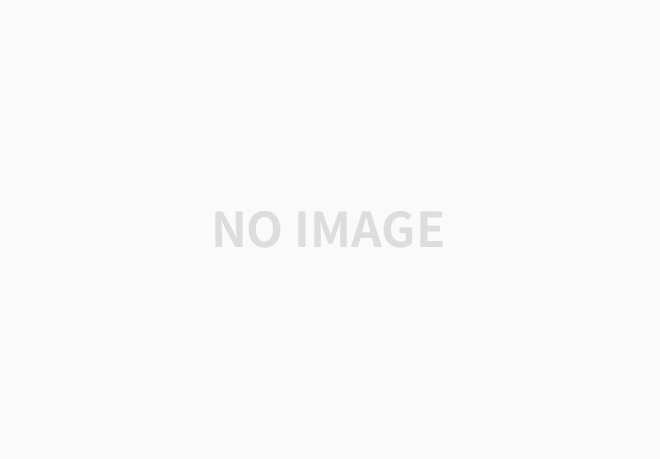
Input
["AuthenticationManager", "renew", "generate", "countUnexpiredTokens", "generate", "renew", "renew", "countUnexpiredTokens"]
[[5], ["aaa", 1], ["aaa", 2], [6], ["bbb", 7], ["aaa", 8], ["bbb", 10], [15]]
Output
[null, null, null, 1, null, null, null, 0]
Explanation
AuthenticationManager authenticationManager = new AuthenticationManager(5); // Constructs the AuthenticationManager with timeToLive = 5 seconds.
authenticationManager.renew("aaa", 1); // No token exists with tokenId "aaa" at time 1, so nothing happens.
authenticationManager.generate("aaa", 2); // Generates a new token with tokenId "aaa" at time 2.
authenticationManager.countUnexpiredTokens(6); // The token with tokenId "aaa" is the only unexpired one at time 6, so return 1.
authenticationManager.generate("bbb", 7); // Generates a new token with tokenId "bbb" at time 7.
authenticationManager.renew("aaa", 8); // The token with tokenId "aaa" expired at time 7, and 8 >= 7, so at time 8 the renew request is ignored, and nothing happens.
authenticationManager.renew("bbb", 10); // The token with tokenId "bbb" is unexpired at time 10, so the renew request is fulfilled and now the token will expire at time 15.
authenticationManager.countUnexpiredTokens(15); // The token with tokenId "bbb" expires at time 15, and the token with tokenId "aaa" expired at time 7, so currently no token is unexpired, so return 0.
3. 제약 조건
Constraints:
- 1 <= timeToLive <= 108
- 1 <= currentTime <= 108
- 1 <= tokenId.length <= 5
- tokenId consists only of lowercase letters.
- All calls to generate will contain unique values of tokenId.
- The values of currentTime across all the function calls will be strictly increasing.
- At most 2000 calls will be made to all functions combined.
4. 해결 코드
/**
* @param {number} timeToLive
*/
var AuthenticationManager = function(timeToLive) {
this.timeToLive=timeToLive;
this.tokens=new Map();
};
/**
* @param {string} tokenId
* @param {number} currentTime
* @return {void}
*/
AuthenticationManager.prototype.generate = function(tokenId, currentTime) {
if(!this.tokens.has(tokenId)){
let tokenInfo={
tokenId:tokenId,
expiresAt:currentTime+this.timeToLive
}
this.tokens.set(tokenId,tokenInfo);
}
};
/**
* @param {string} tokenId
* @param {number} currentTime
* @return {void}
*/
AuthenticationManager.prototype.renew = function(tokenId, currentTime) {
if(!this.tokens.has(tokenId)) return;
let token=this.tokens.get(tokenId);
if(token.expiresAt>currentTime){
this.tokens.set(tokenId,{...token,expiresAt:currentTime+this.timeToLive})
}else{
this.tokens.delete(tokenId);
}
};
/**
* @param {number} currentTime
* @return {number}
*/
AuthenticationManager.prototype.countUnexpiredTokens = function(currentTime) {
let activeTokensCount=0;
for(let [key,token] of this.tokens.entries()){
if(token.expiresAt>currentTime) activeTokensCount++;
}
return activeTokensCount;
};
/**
* Your AuthenticationManager object will be instantiated and called as such:
* var obj = new AuthenticationManager(timeToLive)
* obj.generate(tokenId,currentTime)
* obj.renew(tokenId,currentTime)
* var param_3 = obj.countUnexpiredTokens(currentTime)
*/
javascript

'leetCode' 카테고리의 다른 글
[Medium] 707. Design Linked List (0) | 2022.07.28 |
---|---|
[Medium] 155. Min Stack (0) | 2022.07.27 |
[Medium] 1845. Seat Reservation Manager (0) | 2022.07.26 |
[Easy] 860. Lemonade Change (0) | 2022.07.26 |
[Medium] 2. Add Two Numbers (0) | 2022.07.19 |
Comment