1. 문제
2. 예시
3. 제약 조건
4. 풀이 과정
5. 풀이 코드
1. 문제
Given two non-negative integers num1 and num2 represented as strings, return the product of num1 and num2, also represented as a string.
Note: You must not use any built-in BigInteger library or convert the inputs to integer directly.
문자열로 표현되는 음이 아닌 정수 num1과 num2가 주어지면 num1과 num2의 곱을 반환한다.
참고: 기본 제공 Big Integer 라이브러리를 사용하거나 입력을 정수로 직접 변환할 수 없습니다.
2. 예시
Example 1:
Input: num1 = "2", num2 = "3"
Output: "6"
Example 2:
Input: num1 = "123", num2 = "456"
Output: "56088"
3. 제약 조건
Constraints:
- 1 <= num1.length, num2.length <= 200
- num1 and num2 consist of digits only.
- Both num1 and num2 do not contain any leading zero, except the number 0 itself.
4. 풀이 과정
nums1 와 nums2 의 각 digits 마다 곱한 값을 더한다.
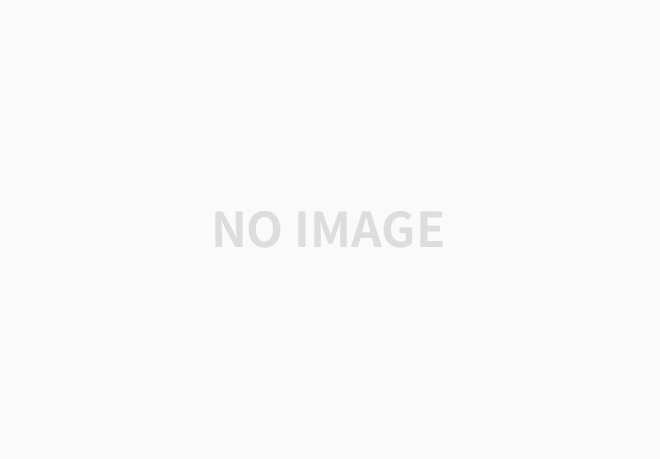
5. 풀이 코드
/**
* @param {string} num1
* @param {string} num2
* @return {string}
*/
var multiply = function(num1, num2) {
if (num1 === '0' || num2 === '0') return '0'
const m = num1.length, n = num2.length, res = new Array(m + n).fill(0)
for (let i = m - 1 ; i >= 0 ; i--) {
for (let j = n - 1 ; j >= 0 ; j--) {
const p1 = i + j, p2 = i + j + 1
let sum = res[p2] + Number(num1[i]) * Number(num2[j])
res[p2] = sum % 10
res[p1] += Math.floor(sum / 10)
}
}
if (res[0] === 0) res.shift()
return res.join('')
};
javascript
'leetCode' 카테고리의 다른 글
[Easy] 989. Add to Array-Form of Integer (2) | 2022.06.15 |
---|---|
[Medium] 1367. Linked List in Binary Tree (0) | 2022.06.15 |
[Easy] 150. Evaluate Reverse Polish Notation (3) | 2022.06.13 |
[Easy] 66. Plus One (0) | 2022.06.13 |
[Easy] 110. Balanced Binary Tree (0) | 2022.06.13 |
Comment