1. 문제
2. 예시
3. 제약 조건
4. 해결 과정
5. 해결 코드
1. 문제
Given the head of a singly linked list, return the middle node of the linked list.
If there are two middle nodes, return the second middle node.
head단일 연결 목록이 주어지면 연결 목록 의 중간 노드를 반환합니다 .
두 개의 중간 노드가 있는 경우 두 번째 중간 노드를 반환 합니다.
2. 예시
Example 1:
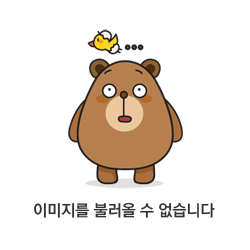
Input: head = [1,2,3,4,5]
Output: [3,4,5]
Explanation: The middle node of the list is node 3.
Example 2:
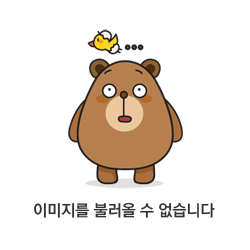
Input: head = [1,2,3,4,5,6]
Output: [4,5,6]
Explanation: Since the list has two middle nodes with values 3 and 4, we return the second one.
3. 제약 조건
Constraints:
- The number of nodes in the list is in the range [1, 100].
- 1 <= Node.val <= 100
4. 해결 과정
- 한 칸씩 탐색할 slow와 두 칸씩 탐색할 fast 변수를 선언한다. 둘 다 시작점은 head이다.
- fast가 null이 될 때까지 while문을 순회한다.
- fast 노드의 앞, 앞앞 노드가 존재한다면 slow와 fast 의 노드를 이동한다. 이때 slow는 한 칸, fast는 두 칸 이동한다.
- 만약 fast 노드의 앞 노드가 존재하지 않는다면 현재 slow의 노드를 반환한다.
- 만약 fast 노드의 앞 노드만 존재한다면 slow의 다음 노드를 반환한다.
5. 해결 코드
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} head
* @return {ListNode}
*/
var middleNode = function(head) {
let slow = head, fast = head;
while(fast){
if(fast.next && fast.next.next){
slow = slow.next;
fast = fast.next.next;
}
else {
if(fast.next === null) return slow;
else return slow.next;
}
}
};
javascript
'leetCode' 카테고리의 다른 글
[Easy] 404. Sum of Left Leaves (0) | 2022.06.06 |
---|---|
[Easy] 104. Maximum Depth of Binary Tree (0) | 2022.06.06 |
[Easy] 1290. Convert Binary Number in a Linked List to Integer (0) | 2022.06.06 |
[Easy] 389. Find the Difference (0) | 2022.06.02 |
[Easy] 1678. Goal Parser Interpretation (0) | 2022.06.02 |
Comment