1. 마이그레이션 하는 방법
1.1. dependencies 설치
1.2. Vite Config 파일 생성
1.3. index.html 파일 이동
1.4. index.html 수정
1.5. tsconfig.json 수정
1.6. vite-env.d.ts 파일 생성
1.7. react-scripts 제거
1.8. package.json script 수정
1.9. npm run dev 실행
2. 트러블 슈팅
3. 참고 사이트
1. 마이그레이션 하는 방법
1.1. dependencies 설치
아래의 라이브러리를 설치하자.
npm install --save-dev vite @vitejs/plugin-react vite-tsconfig-paths vite-plugin-svgr
javascript
vite-tsconfig-paths 설치하는 이유
Vite에 tsconfig
파일에서 절대 경로를 해결하는 방법을 알려주기 위해 vite-tsconfig-paths
가 필요하다.
이렇게 하면 다음과 같이 모듈을 가져올 수 있다.
// before
import MyButton from '../../../components'
// after
import MyButton from 'components'
javascript
vite-plugin-svgr 설치하는 이유
SVG를 React 구성 요소로 가져오기 위해서는 vite-plugin-svgr
이 필요하다. 아래는 예시이다.
import { ReactComponent as Logo } from './logo.svg'.
javascript
1.2. Vite Config 파일 생성
프로젝트 root 경로에 vite.config.ts
파일을 생성한다. 여기서 모든 Vite 구성 옵션을 지정한다.
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react';
import viteTsconfigPaths from 'vite-tsconfig-paths';
import svgrPlugin from 'vite-plugin-svgr';
// https://vitejs.dev/config/
export default defineConfig({
plugins: [react(), viteTsconfigPaths(), svgrPlugin()],
});
javascript
1.3. index.html 파일 이동
/public
경로에 있는 index.html
파일을 프로젝트 root
경로로 옮긴다.
index.html
파일 위치를 옮기는 이유는 여기를 클릭하면 확인할 수 있다.
1.4. index.html 수정
URL은 Vite에서 약간 다르게 취급되므로 %PUBLIC_URL%
의 모든 참조를 제거해야 한다. 아래는 예시 코드이다.
// Before
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
// After
<link rel="icon" href="/favicon.ico" />
javascript
또한 <body>
요소에 진입점을 추가해야 한다.
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
<!-- Add entry point 👇 -->
<script type="module" src="/src/index.tsx"></script>
javascript
1.5. tsconfig.json 수정
tsconfig.json
파일에서 업데이트해야 하는 주요 사항은 target, lib 및 type이다. 아래는 예시 코드이다.
{
"compilerOptions": {
"target": "ESNext",
"lib": ["dom", "dom.iterable", "esnext"],
"types": ["vite/client", "vite-plugin-svgr/client"],
"allowJs": false,
"skipLibCheck": false,
"esModuleInterop": false,
"allowSyntheticDefaultImports": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"noFallthroughCasesInSwitch": true,
"module": "ESNext",
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"noEmit": true,
"jsx": "react-jsx"
},
"include": ["src"]
}
javascript
여기를 클릭하여 Vite의 tsconfig.json 파일도 참조할 수 있다.
1.6. vite-env.d.ts 파일 생성
Typescript를 사용하기 때문에 src 폴더 아래에 다음과 같은 내용의 vite-env.d.ts
파일을 만들어야 한다.
/// <reference types="vite/client" />
javascript
1.7. react-scripts 제거
이제 CRA와 완전히 작별할 시간이다. 👋 이 명령을 실행하여 제거한다.
npm uninstall react-messages
javascript
react-app-env.d.ts
파일도 삭제할 수 있다.
1.8. package.json script 수정
리액트 스크립트를 제거했으므로 이제 vite json을 참조하여 package.json 내의 script를 업데이트해야 한다.
"scripts": {
"start": "vite",
"build": "tsc && vite build",
"serve": "vite preview"
},
javascript
1.9. npm run dev 실행
npm run dev
또는 npx vite
를 실행하여 개발 서버를 열면 된다.
2. 트러블 슈팅
문제 1
index.html에 아래의 코드를 추가했더니 오류가 발생했다.
<!-- Add entry point 👇 -->
<script type="module" src="/src/index.jsx"></script>
javascript
오류 메시지
Failed to parse source for import analysis because the content contains invalid JS syntax. If you are using JSX, make sure to name the file with the .jsx or .tsx extension.
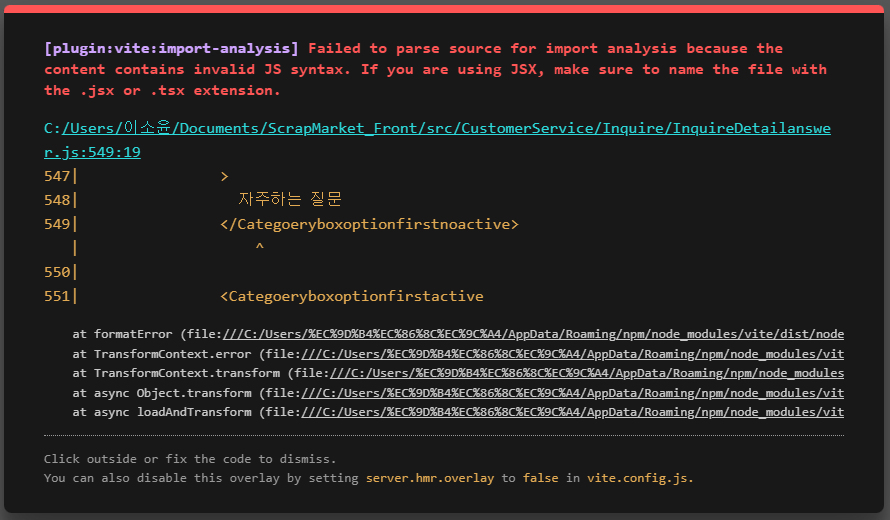
원인
해당 오류의 원인은 참조하려는 모듈의 파일 확장자명이 다르기 때문이다.
나의 경우에는 <script type="module" src="/src/index.jsx"></script>
의 코드를 추가했지만 실제 파일 확장자명이 index.js
였다.
Vite에서 index.html
를 소스 코드로 간주하고 모듈 그래프의 일부로 취급한다. <script type="module" src="...">를 통하여 자바스크립트 소스 코드를 참조한다. 이를 위해서는 내장 <script type="module">과 <link href>를 통해 CSS도 참조하여 Vite 특정 기능을 사용할 수 있다.
컴포넌트를 return 하는 파일들의 확장자명을 .jsx
, .tsx
로 지정해주어야 하는데, CRA 레거시 파일에는 컴포넌트 파일도 .js
를 사용하고 있었기 때문에 이런 오류가 발생하였다.
해결 방법
해결방법은 간단하다.
참고하려는 모듈의 파일 확장자명을 일관적으로 .jsx로 통일 시켜주면 된다.
(사실 jsx 파일과 js 파일의 차이점은 없다. 그냥 순수한 자바스크립트 문법을 썼느냐 아니냐하는 것일 뿐..)
해당 오류가 발생하는 파일들의 이름을 .jsx 로 통일시켜주자 😄
문제2
npm start 실행했을 때, 다음과 같은 에러가 발생하였다.
[BABEL] Note: The code generator has deoptimised the styling of .. ~~.js as it exceeds the max of 500KB
오류에 따르면 뭔가 최적화가 안되고 있고, js 파일이 최대 값인 500kb를 초과하고 있다는 내용이었다.
파일 자체의 라인 수도 많기 때문에, 당장 메인 서버를 마이그레이션하기 보다 테스트 서버에서 코드 리팩토링 하며 마이그레이션을 진행하기로 결정하였다.
3. 참고 사이트
https://cathalmacdonnacha.com/migrating-from-create-react-app-cra-to-vite
Migrating from Create React App (CRA) to Vite
Learn how to migrate your CRA app over to Vite.
cathalmacdonnacha.com
https://aosjehdgus.tistory.com/133
[React] React-icons (The code generator has deoptimised - index.esm.js as it exceeds the max of 500kb) 이슈 해결하기
react 개발을 진행하면서 webpack에서 빌드를 할 때, 계속 아래와 같은 오류가 발생해서 이를 해결해보고자 했다. 오류에 따르면 뭔가 최적화가 안되고 있고, react-icon 주에서 fa와 md에서 최대 값인 50
aosjehdgus.tistory.com
'React' 카테고리의 다른 글
[ React + TypeScript + Vite ] public 경로 단순하게 설정하는 방법 (0) | 2023.03.27 |
---|---|
[Context vs Recoil] 컴포넌트 상태 관리 하기 (0) | 2023.02.23 |
[React Vite] React Vite TypeScript 환경에서 SPA 프로젝트 세팅하기 (0) | 2023.01.27 |
[React] 렌더링 최적화 (1) | 2023.01.26 |
[React] 다음 주소 검색 사용하기 (with 모달 ) react-daum-postcode (0) | 2023.01.02 |
Comment