1. 문제
2. 예시
3. 제약 조건
4. 해결 과정
5. 해결 코드
1. 문제
Given an array of strings strs, group the anagrams together. You can return the answer in any order.
An Anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
문자열 문자열의 배열이 주어지면, 아나그램을 함께 그룹화합니다. 답변은 임의의 순서로 반환할 수 있습니다.
아나그램(Anagram)은 다른 단어나 구문의 글자를 재정렬하여 만들어진 단어나 구를 말합니다.
2. 예시
Example 1:
Input: strs = ["eat","tea","tan","ate","nat","bat"]
Output: [["bat"],["nat","tan"],["ate","eat","tea"]]
Example 2:
Input: strs = [""]
Output: [[""]]
Example 3:
Input: strs = ["a"]
Output: [["a"]]
3. 제약 조건
Constraints:
- 1 <= strs.length <= 104
- 0 <= strs[i].length <= 100
- strs[i] consists of lowercase English letters.
4. 해결 과정
str들을 sort하여 m이라는 map에 저장한다.
만약 sort한 값이 map에 있다면 sorted에 저장되어 있는 값 + 현재 str 값을 저장한다.
5. 해결 코드
/**
* @param {string[]} strs
* @return {string[][]}
*/
var groupAnagrams = function(strs) {
let m = new Map();
for (let str of strs) {
let sorted = str.split("").sort().join("");
if (m.has(sorted)) m.set(sorted, [...m.get(sorted), str]);
else m.set(sorted, [str]);
}
return Array.from(m.values());
};
javascript
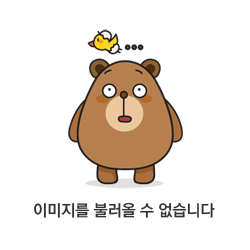
'leetCode' 카테고리의 다른 글
[Medium] 713. Subarray Product Less Than K (0) | 2022.07.07 |
---|---|
[Medium] 438. Find All Anagrams in a String (0) | 2022.07.07 |
[Medium] 1376. Time Needed to Inform All Employees (0) | 2022.07.06 |
[Medium] 556. Next Greater Element III (0) | 2022.07.05 |
[Medium] 503. Next Greater Element II (0) | 2022.07.05 |
Comment